How to Add Google Maps to Your Next.js App
Google Maps is a mapping service provided by Google. It is a map of the world and is used to find places. Before the internet, finding places or planning travel required paper maps, asking for directions, and road signs. Those days are gone, Google Maps is one of the most popular navigation apps used by billions of users worldwide. In this blog post it will be about how to add Google Maps to your Next.js app.
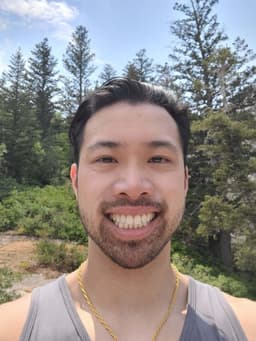
Jordan Wu
5 min·Posted
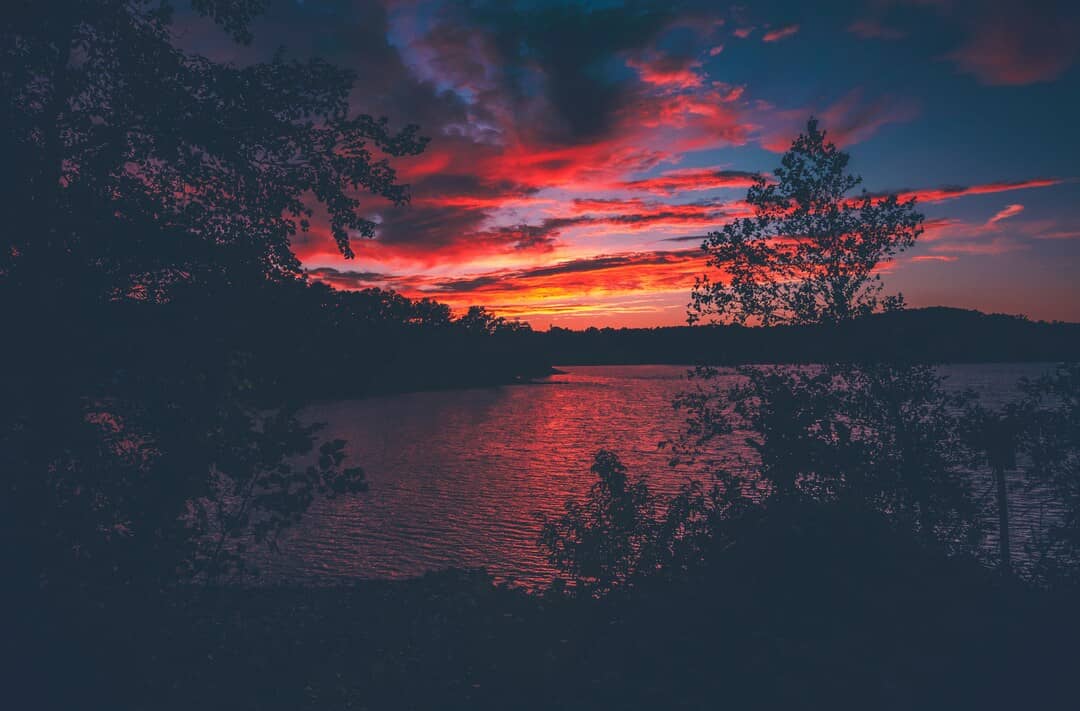
Table of Contents
What is Google Maps?
Google Maps is a map on the web. It is used to find places and give you directions. It is continuously updated with data like real time traffic and business information. All you need is a device with the internet to use it. Having an accurate map of the world is such a useful tool that many companies use Google Maps as part of their business, like ride sharing apps, real estate apps, delivery apps, social media apps, games apps like geoguessr, etc. Google Maps is such a useful tool for software companies to utilize in their platform.
How to Add Google Maps to Next.js App Router
There are many ways to add Google Maps to your Next.js app. The approach I went with is using a newer library called React Google Maps that is currently being maintained and has all the features I need. The other libraries were much older and were not maintained but widely used.
Create Google Maps API Key
You would need to set up an account on the Google Maps Platform.
- In the Google Cloud Console, on the project selector page, click Create Project to begin creating a new Cloud project.
- Make sure that billing is enabled for your Cloud project. Google Maps Platform features a recurring $200 monthly credit.
- See the Google Maps Platform APIs and SDKs that you can enable by going clicking on the project you created.
- Go to the Keys & Credentials page and select your project.
- On the Credentials page, click Create credentials > API key. The API key created dialog displays your newly created API key.
- Click Close. The new API key is listed on the Credentials page under API keys.
- Click on new API key and add website restrictions to only allow your domain access to the API key, for example
*.example.com/*
. Click Save.
Now that you have the API key you would need to add it to your local .env.local
file.
NEXT_PUBLIC_GOOGLE_MAPS_API_KEY="GOOGLE_MAPS_API_KEY"
Be sure to add the environment variable to your cloud deployment platform, for me that will be Vercel, Declare an Environment Variable.
Setup
Install the following dependencies:
pnpm add @vis.gl/react-google-maps
@vis.gl/react-google-maps
is a React library for Google Maps JavaScript API. It provides a collection of React components and hooks for you to customize Google Maps on your website and is a reactive wrapper for the Google Maps JavaScript API while still leaving the map instance in charge of state updates. Meaning Google Maps is a component that should manage its own state without you having to manage any state. For example you should not be controlling the map from mouse, pointer and touch events. It should be handled by Google Maps.
Create Google Maps Playground Page
Add a Google Maps playground page to your Next.js App. Create page.tsx
component.
'use client'
import { APIProvider, Map, Marker } from '@vis.gl/react-google-maps'
export default async function ChatPlaygroundPage() {
const apiKey = process.env.NEXT_PUBLIC_GOOGLE_MAPS_API_KEY as string
return (
<APIProvider apiKey={apiKey}>
<Map
style={{ width: '100%', height: '100%' }}
defaultCenter={{ lat: 38.73583285264431, lng: -109.59319053900342 }}
defaultZoom={12}
gestureHandling={'greedy'}
disableDefaultUI={true}
/>
<Marker
position={{ lat: 38.73583285264431, lng: -109.59319053900342 }}
/>
</APIProvider>
)
}
This will add Google Maps with a marker on Arches National Park in Utah! This is just a simple map and you can add more features like map styles, place autocomplete, place details, street view, directions, etc. If you want to learn more check out Google Maps Platform Documentation.
Check out Google Maps Playground
Summary
Google Maps is such a useful tool to have on your website. The platform provides developers with business information, ratings, reviews and more for over 200 million businesses and places around the world. It's a great solution if you need to store business information such as their address and geolocation on your platform. With its Autocomplete service you can type in the name of the place or the address of the place and view it on the map instantly. In this blog post you added Google Maps to your Next.js app.